How To Nest Ternaries
Before we talk about ternaries let's take a quick look at if statements. Ignoring the indentation, do you write if statements like this?
int result=0;
if (cond1)
if (cond2)
result = 2;
else
result = 10;
Of course not. We use braces. Can you tell me which if that else belongs to?
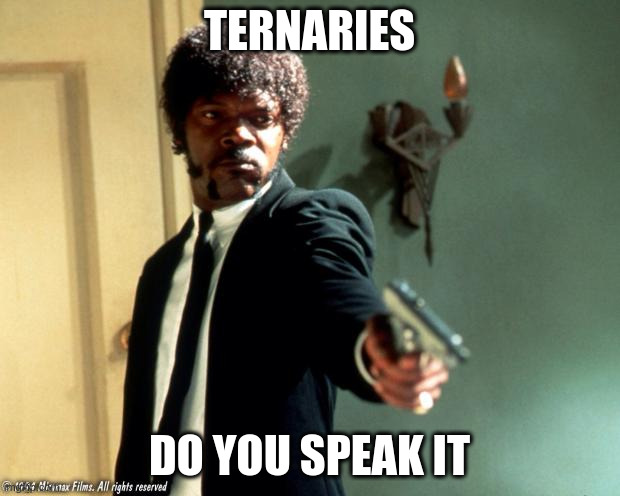
How often do you see an if statement written in this ridiculous way? Pretty much never. Let's apply the same problem and problem solving to ternaries. Here's that same if statement which you should equally never write.
int result = cond1 ? cond2 ? 2 : 10 : 0;
If you didn't know, the else is on the innermost if. Here's how people normally write that if, the braces and spacing make it more clear.
int result = 0;
if (cond1) {
if (cond2) {
result = 2;
} else {
result = 10;
}
}
Here is how it might look as a nested ternary but I would never write it this way.
int result = cond1 ? (cond2 ? 2 : 10) : 0;
Ternaries can't have curly braces but can have parenthesis. Let's work through how to make it better by looking at different if statements. Is this better than the original if statement?
int result = 0;
if (cond1 && cond2) {
result = 2;
} else if (cond2) {
result = 10;
}
Some people may dislike that cond2 is used twice. I prefer the next style and I always leave a comment on empty if statements to show it was intentional.
int result = 0;
if (!cond1) {
// Nothing
} else if (cond2) {
result = 2;
} else {
result = 10;
}
Is this the best of the three? The else statements always go at the end, the if statements are flat. Let's apply this to ternaries. This I would write.
int result = !cond1 ? 0 : cond2 ? 2 : 10;
If the ternaries look like the below you did it right
tern(cond1, true1, cond2, true2, ..., condN, trueN, falseValue)
Let's try an example where it isn't flat.
int result = 0;
if (cond1) {
if (cond2) {
result = 2;
} else {
result = 10;
}
} else {
if (cond2) {
result = 50;
} else {
result = 80;
}
}
Here's my solution, and I'll get rid of the literals to make it look more like real code.
int result = cond1 ? ( (cond2) ? two : ten ) : cond2 ? fifty : eighty;
How easy is it to see the inner ternary? It's ridiculously easy.
- We're forced to use parenthesis, but I put spaces around it to make it easier to notice.
- Since the conditional is inside the true part of another ternary, I want it to be clear it's a conditional so I write it in params. I may do this for conditionals on the right when it's more than a single compare.
Mind you this is how I would write it if I had a good reason. Using grep on my current codebase I see I have dozens of nested ternaries, but they all follow the style of having it on the right side.